7. Audio playback - Pygame¶
Among LubanCat-RK series boards, LubanCat 2 and LubanCat 1 have audio rk809-codec. Through the audio codec chip. In this chapter, we will use the music module of the Python-Pygame library to write test codes for audio playback experiments on the lubancat board.
7.1. Introduction to the Pygame library¶
Pygame is a tool for developing games in the Python library. Perhaps it is a bit restrictive to use Pygame. Pygame can be used not only for game development, but also for display processing, multimedia device processing, etc. The library provides many multimedia and display device operation functions.
7.2. Experiment preparation¶
7.2.1. Sound resources on the board¶
Take LubanCat 2 as an example:
# Enter the following command in the terminal to view the display device resources:
ls /dev/snd/
Check the audio device resource example on the board, just for reference:
cat@lubancat:~/sound$ ls /dev/snd/
by-path controlC0 pcmC0D0c pcmC0D0p seq timer
Among them, controlC0 is used for sound card control, such as channel selection, microphone control, etc. pcmC0D0c is a pcm device for recording, pcmC0D0p is a pcm device for playback, seq is a sequencer, and timer is a timer.
For more information about the Lubancat audio system in linux, please refer to “Audio” .
7.2.2. Hardware connection¶
LubanCat 1 and LubanCat 2 boards have earphone + microphone interface, just connect the earphone or other playback devices to the Lubancat interface.
7.2.3. Pygame library installation¶
We use the apt tool to install.
# Enter the following command in the terminal to install the Pygame library:
sudo apt -y install python3-pygame
7.2.4. The Pygame library uses¶
After installing the corresponding library, we can use the installed Pygame library to write some test code.
7.3. Test code¶
测试代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | """ pygame audio playback test """
import sys
import time
import threading
if len(sys.argv) == 2:
m_filename = sys.argv[1]
else:
print(
"""Instructions:
python3 sound_test.py <music_name.mp3>
example: python3 sound_test.py test.mp3"""
)
sys.exit()
import pygame
exitright = 'e'
def get_quitc():
'Get exit character'
# Global variable
global exitright
# Get user input
exitright = input("After entering the letter q, press enter to exit playback\n")
try:
# Initialize the audio device
pygame.mixer.init()
# Check audio device initialization
if pygame.mixer.get_init() is None:
raise RuntimeError('The audio resource was not properly initialized, please check if the audio device is available',)
# Load audio files, mp3 files are not played here, because pygam's playback support for MP3 format is limited
pygame.mixer.music.load(m_filename)
# Set the volume to 50%
pygame.mixer.music.set_volume(0.2)
# Play audio file 1 time
pygame.mixer.music.play(0)
# Start the child thread, waiting for the user to enter the exit character
quitthread = threading.Thread(target=get_quitc,daemon=True)
quitthread.start()
# Get the audio playback status, if it is playing, wait for the playback to finish
while pygame.mixer.music.get_busy():
# Idle delay, release cpu
if exitright in ('q','Q'):
raise
time.sleep(1)
except pygame.error as e:
print("An exception occurred while executing the program: ",e,"\nWaiting for the audio resource to be released")
# Try to stop playback
pygame.mixer.music.stop()
# Wait for an audio resource to be free
while pygame.mixer.music.get_busy():
time.sleep(1)
finally:
# Uninstall the initialized audio device
print("Quit playing")
pygame.mixer.quit()
sys.exit()
|
The code function has been given in detail in the comments.
7.3.1. Experimental procedure¶
Before testing the code, please place the test audio files of various formats in the same directory as the code in the io/sound directory of the supporting code folder, and then run the program.
The test code plays a user-specified audio file.
# Enter the following command in the terminal:
sudo python3 sound_test.py test.mp3
# or
sudo python3 sound_test.py test.wav
# or
sudo python3 sound_test.py test.ogg
There are a lot of resources loaded when the pygame library is imported, so it will take a while for the experimental phenomenon to appear. After the program runs normally, you can hear the audio played on the playback device~
During the music playing process, if you want to exit, please operate according to the prompt information printed by the terminal, enter q in the terminal and press Enter, and the program will end. If the program terminates abnormally, the audio device resources will be occupied, and the development board needs to be restarted before it can be used again.
Running shows:
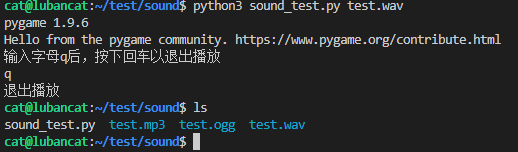
7.3.2. Reference¶
For more information about the use of the Python Pygame library, please refer to Pygame Tutorial .
About Pygame music, please refer to Pygame music.