3. Run python¶
This chapter explains how to use Python on the Lubancat board, and its usage is no different from that on the PC.
3.1. Use the python terminal interactive environment¶
We can directly run python commands to enter Python interactive programming. Interactive programming does not require the creation of script files, and codes are written through the interactive mode of the Python interpreter.
You can do some simple operations in the interactive environment, such as outputting “hello world!” in the terminal, doing some arithmetic operations, etc., and finally exit through exit()
:
# Type in terminal
python3
# Output content
Python 3.8.10 (default, Jun 22 2022, 20:18:18)
[GCC 9.4.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
# Enter and press enter
>>> print("hello world!")
# Output
hello world!
>>>
# Output
>>> 8+2
# Output
10
# To exit the interactive mode, you can also use quit() or the key combination Ctrl+D to exit
>>> exit()
cat@lubancat:~$
Alternatively, to use python in the terminal, you can also install ipython (enhanced interactive Python shell):
# Install ipython
sudo pip3 install ipython
#Use
cat@lubancat:~$ ipython
Python 3.8.10 (default, Jun 22 2022, 20:18:18)
Type 'copyright', 'credits' or 'license' for more information
IPython 8.6.0 -- An enhanced Interactive Python. Type '?' for help.
In [1]:
# Enter 8+2
In [1]: 8+2
Out[1]: 10
In [2]:
#Use exit to exit the interaction
3.2. Use python script¶
We can also write a simple Python code, save it as hello.py
file and execute it.
You can use the serial port to log in to the board system and use the vim editor to write, or vscode ssh to log in to the board for writing:
The content of the hello.py code file is as follows:
print("Hello LubanCat!")
Then run:
# Write hello.py
# Use the ls command to confirm that the file exists in the directory
ls
# The following is output
hello.py
# Run
python3 hello.py
# Output
Hello LubanCat!
The following is the scene of writing and running using VS Code Remote:
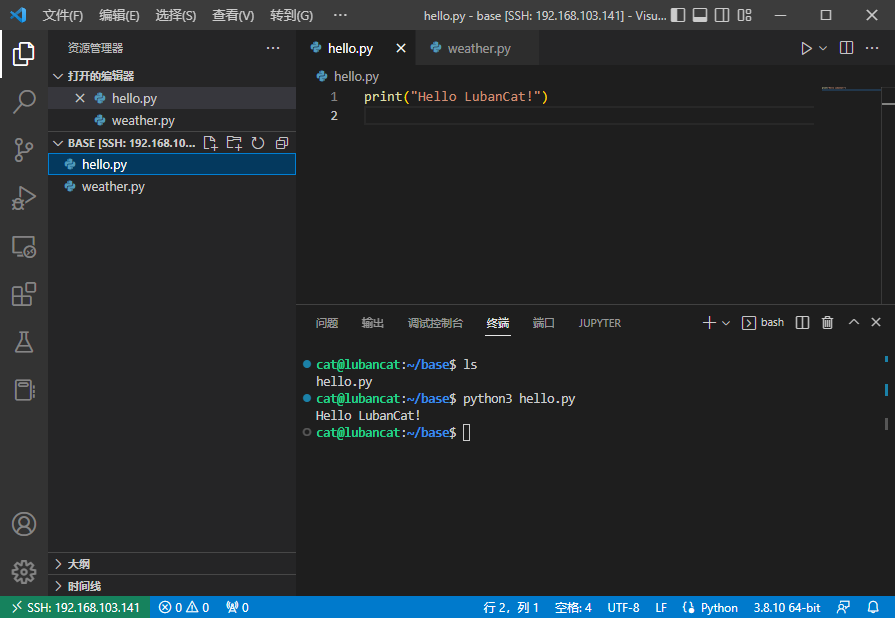
Use script, you can also specify the path of the interpreter in the script, such as:
.. code-block:: python
- caption
base/hello.py file content
#!/usr/bin/python print(“Hello LubanCat!”)
Then run:
# Run
cat@lubancat:~$ ./hello.py
Hello LubanCat!
cat@lubancat:~$
3.3. Use python IDE¶
If you use a desktop system and screen display, you can choose to install the Python IDE for learning. Take the THONNY IDE as an example:
#Use the APT package manager to install
sudo apt install thonny
Open the display after installation (take the XFCE4 desktop as an example):
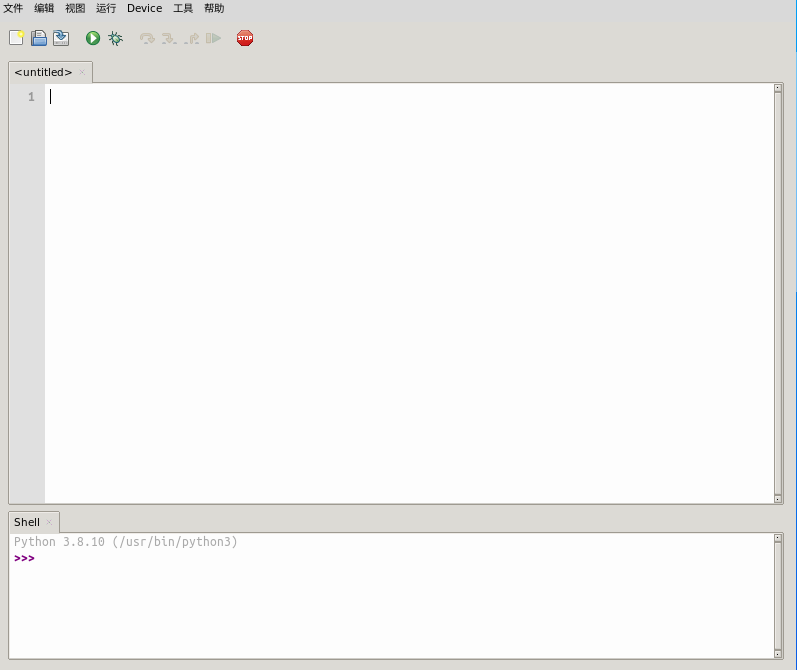
Test, click Run:
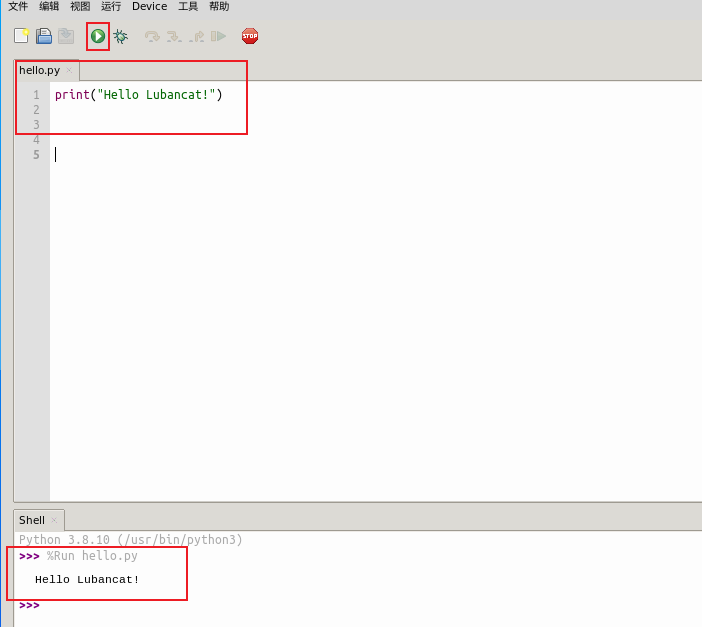
Thonny is an integrated development environment (IDE) for Python beginners. It supports display grammatical errors and interpretation of scope, built -in debugger and gradual execution evaluation, and GUI interface to manage Python software packages.
3.4. Import other software packages¶
In addition to the Python code library of running foundation, it can also import third -party software packages in the code to run more complex applications.
To explain the example program, we create a new `` Get_info.py``, write the following code:
#Import psutil package
import psutil
import datetime
#Get the current user information, the output name username, Terminal terminal, host host address, Started login time, PID process ID.
print("Current user:", psutil.users())
#Get the current system time and convert it to a natural time format
print("Current System Time:", datetime.datetime.fromtimestamp(psutil.boot_time()).strftime("%Y-%m-%d %H: %M: %S"))
print("\n-----------Memory information-------------------")
mem = psutil.virtual_memory()
print("System memory all information:", mem)
mem_total = float(mem.total)
mem_used = float(mem.used)
mem_free = float(mem.free)
mem_percent = float(mem.percent)
print(f"Total memory:{mem_total}")
print(f"The system has used memory:{mem_used}")
print(f"System free memory:{mem_free}")
print(f"System memory usage rate:{mem_percent}")
print("\n-----------CPU information-------------------")
print("CPU summary information:", psutil.cpu_times())
print("CPU logic number:", psutil.cpu_count())
print("CPU frequency:", psutil.cpu_freq())
注解
Python’s PSUTIL (Process and System Utilities) library is a cross -platform third -party library that can easily realize the process and system utilization of system operation (including CPU, memory, disk, network, process, etc.). It is mainly used for system monitoring, analysis, and restricting the management of system resources and processes.
Save the file and try to run the get_info.py program:
# Runtime
python3 get_info.py
# Output
Current user:[suser(name='cat', terminal='ttyFIQ0', host='', started=1662631296.0, pid=742)]
Current System Time: 2022-11-01 16: 28: 20
-----------Memory information-------------------
System memory all information:svmem(total=2059280384, available=892481536, percent=56.7, used=1123770368, free=30068736, active=1374724096, inactive=373829632, buffers=83066880, cached=822374400, shared=17391616, slab=232140800)
Total memory:2059280384.0
The system has used memory:1123770368.0
System free memory:30068736.0
System memory usage rate:56.7
-----------CPU information-------------------
CPU summary information: scputimes(user=2365.82, nice=3.25, system=765.33, idle=335360.41, iowait=79.58, irq=0.0, softirq=50.22, steal=0.0, guest=0.0, guest_nice=0.0)
CPU logic number: 4
CPU frequency: scpufreq(current=1992.0, min=408.0, max=1992.0)
For some packaged packages, we can install in the following way (take the requests software package as an example):
# Method 1: Use PIP to install directly
sudo pip3 install requests
# Method 2: Use APT to install
sudo apt -y install python3-requests
Some dependencies are also supported by Debian, so you can use APT to install directly. For standard dependencies, we recommend using APT installation. The installation method of PIP is mainly used to install a third -party software package that cannot be found in APT.
If you use Thonny, you can click the tools of the interface-> management package, query and install the software package:
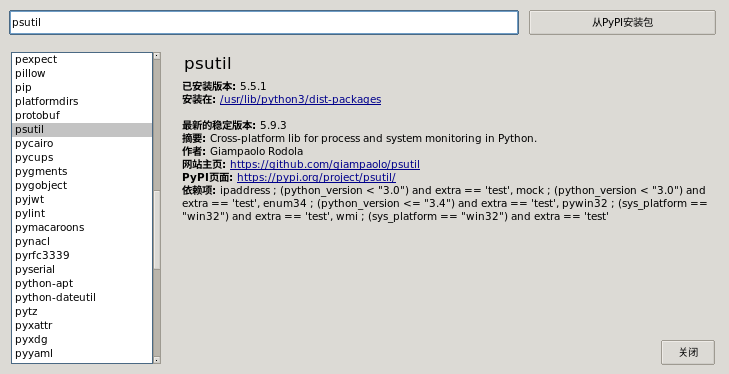